I had a request to take a servo and attach it to the Arduino. Watch the video, I know the text is hard to read in the video. Please see full code below. I added some extra things to make sure the servo can’t be pushed to go beyond the limit because the servo I am using can only go 180. Some servos can go 360 degrees. I also added some code check if someone is pushing both buttons at once.
Parts Needed |
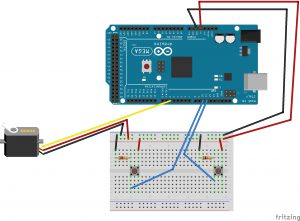
#include <Servo.h> Servo myservo; // create servo object to control a servo const int LeftbuttonPin = 10; //Left button const int RightbuttonPin = 11; //Right button int pos = 90; //used to keep track of the position of the servo int LeftState = 0; //left is not pressed int RightState = 0; //right is not pressed void setup() { myservo.attach(2); // attaches the servo on pin 2 to the servo object pinMode(LeftbuttonPin, INPUT); //make the pin an input pinMode(RightbuttonPin, INPUT); //make the pin an input myservo.write(90); //move to 90 first or middle Serial.begin(9600);//setup serial for diagnostics } void loop() { LeftState = digitalRead(LeftbuttonPin); //grab button value high or low RightState = digitalRead(RightbuttonPin); //grab button value high or low if (LeftState == HIGH && RightState == HIGH) { // do nothing can't go in both directions Serial.println("both pushed"); } else { if(LeftState == HIGH) //check if left pushed { Serial.println("left high"); if(pos < 180) { Serial.println("left not at end"); pos += 1; //increase position by 1 Serial.println(pos); myservo.write(pos); } } if(RightState == HIGH) //check if right pushed { Serial.println("right high"); if(pos > 0) { Serial.println("right not at end"); pos -= 1; // decrease position by 1 Serial.println(pos); myservo.write(pos); } } } // tell servo to go to position in variable 'pos' delay(50); // waits 15ms for the servo to reach the position }